Getting started
Learn how to get started with Croct.
In this guide, we will walk you through setting up your account, creating your first component, and implementing a personalized hero for a fictional startup.
We have divided this guide into two parts to accommodate readers with different backgrounds. The first part is for a general audience and covers the first steps of setting up the platform. The second part is a hands-on tutorial for developers with basic knowledge of HTML and JavaScript.
Regardless of your background, we recommend reading the first part to get a better understanding of the platform's concepts and how they relate to each other.
Let's get started!
Get into your account
Before we begin, you will need to create an account if you have not already.
Click here to create a free account and get started.
Next, open our admin application and sign in using your login details. You will then see your home page showing all the organizations you have access to:
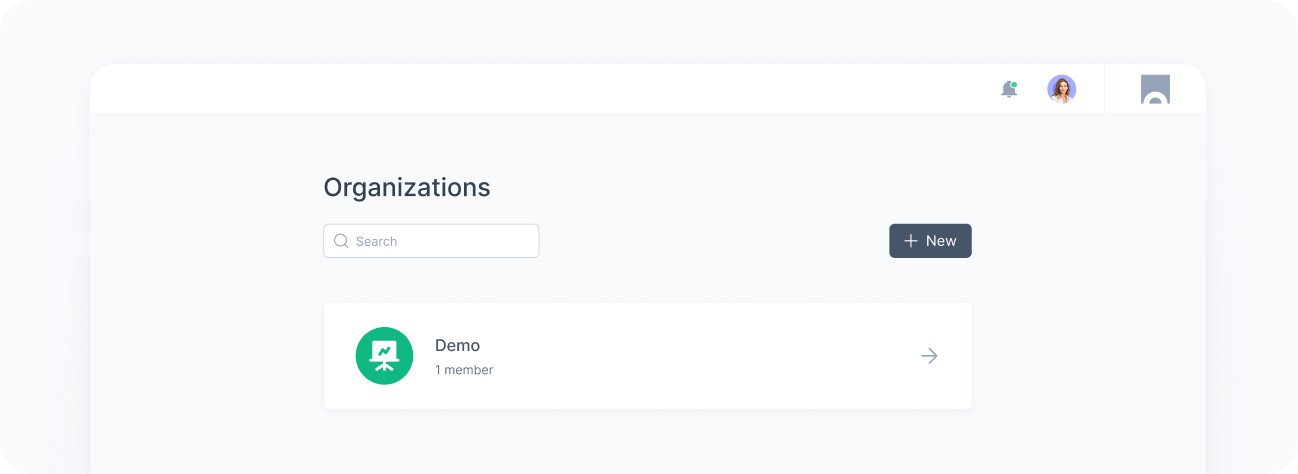
First-time users will see the demo organization with sample components and slots. Feel free to explore and get a feel for the platform.
Set up an organization
Our platform is built around a hierarchical structure that makes it easier for you to control and manage data. Each level gives you control over different aspects of your data, such as access policies, data security, and privacy settings.
Here is a breakdown of the platform's hierarchy:
- Organization
Reflects real-world organizations or accounts for personal use. - Workspace
Represents organizational divisions, such as business units, projects, or environments. - Application
Refers to the specific websites or apps you want to personalize.
For now, we will only cover the essential information you need to know to get started. To learn more, please refer to the Hierarchy overview.
Let's create your organization:
Click the + New button in the center right of the page.
Select whether your organization is a Business or Personal account.
Enter your organization's name and other details.
Click Save.
Next, create your first workspace:
Click the + New button in the center of the page.
Enter your workspace's name and other details.
If your company does not have divisions, you can use the same name as your organization.
Click Save.
Lastly, create an application:
Click Applications in the left sidebar.
Click the + New button in the center of the page.
Enter your application's name and other details.
Under Environment, select Development.
Development applications have more restrictive security settings to ensure your data is safe. Before you go live, you will need to create a production application.
Click Save.
Now copy your Application ID, as we will need it later.
Create your first slot
Before creating your first slot, let's explain some basic concepts.
Components are the building blocks for applications that ensure consistent design and reusability. You can even nest them to create more complex structures, such as a hero section that incorporates a CTA component.
Designers can think of components like Figma's components, while developers can view them as UI elements.
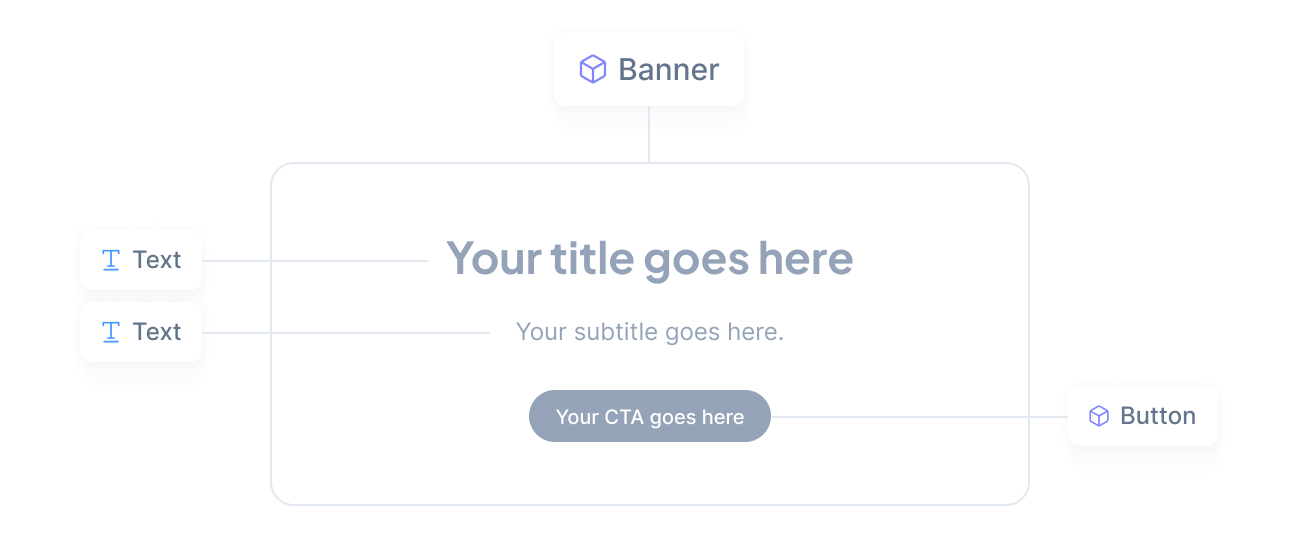
Slots, on the other hand, are the placeholders for components. They can display static or personalized content:
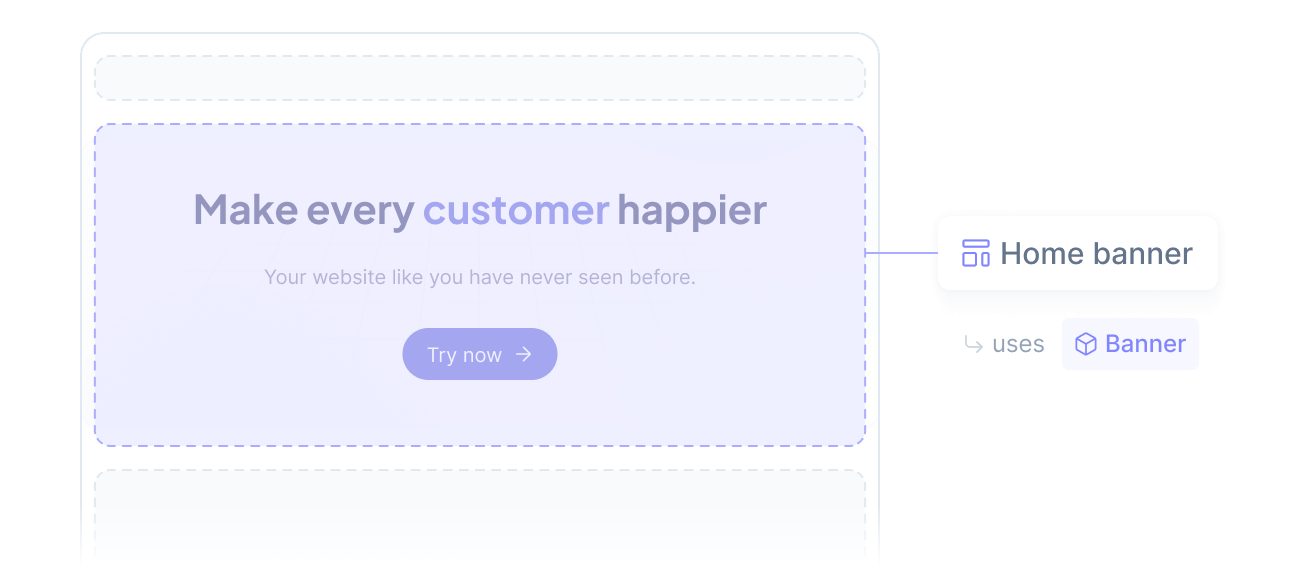
Each slot is linked to a single component that defines its structure. For example, a homepage hero and a landing page hero can both be linked to the same hero section component to ensure visual consistency.
In our example, we will create a component for the hero banner and a slot for the homepage hero.
This is the schema for our hero component:
{"type": "structure","attributes": {"title": {"label": "Title","description": "The title of the hero.","type": {"type": "text"}},"subtitle": {"label": "Subtitle","description": "The subtitle of the hero.","type": {"type": "text"}},"cta": {"type": {"type": "structure","title": "CTA","description": "The call-to-action button.","attributes": {"link": {"label": "Link","description": "The destination URL.","type": {"type": "text","format": "url"}},"label": {"label": "Label","description": "The call-to-action label.","type": {"type": "text"}}}}}}}
The schema structure is already covered in the Schema reference, so we will not delve into it here.
For now, let's focus on creating our first component:
Click the name of your workspace in the top left of the page to go back to the workspace home.
Click Components in the left sidebar.
Click the + New button in the center of the page, and select Regular component.
Enter the Hero banner as the component name, hero-banner as the component ID, and paste the above JSON in the Schema field.
Click Save.
Now we have everything we need to create our first slot:
Click Slots in the left sidebar.
Click the + New button in the center of the page.
Enter the Homepage hero as the slot name, homepage-hero as the slot ID, and select the Home banner component.
Click the pencil icon () on the right of the Default content to edit its content.
Download the default content to save some typing.
Click the options icon () on the top right of the Homepage hero and click the Import content button.
Select the default-content.json file you downloaded in the previous step.
Click Save.
Integrate the SDK
Before we can implement our new component, we need to integrate Croct in our application. The details vary slightly depending on your stack of choice, but the overall process is the same.
We strongly recommend using a package manager like NPM or Yarn for a better developer experience, but you can also install the SDK directly from our CDN.
Choose one of the options below to get started:
<script src="https://cdn.croct.io/js/v1/lib/plug.js"></script>
Then, initialize the SDK with the application ID you copied earlier:
<script>// Replace with your application IDcroct.plug({appId: 'YOUR_APPLICATION_ID'});</script>
Implement your new slot
Time to dive into the exciting part! Let's get our hands dirty, write some code, and see our new slot in action.
First, let's define the markup that will give form to our component:
<div id="hero-banner"><h1>Your static title</h1><p class="subtitle">Your static subtitle.</p><a class="cta" href="#">Your static CTA</a></div>
Then, let's fetch the slot and render it:
<script>croct.fetch('homepage-hero').then(({content}) => {const banner = document.getElementById('hero-banner');const title = banner.querySelector('h1');const subtitle = banner.querySelector('.subtitle');const cta = banner.querySelector('.cta');title.textContent = content.title;subtitle.textContent = content.subtitle;cta.textContent = content.cta.label;cta.href = content.cta.link;});</script>
While the focus of this tutorial is not on styling the component, your new component surely deserves some love. You can style it using your preferred CSS framework or simply add the following snippet to your application:
1<link rel="stylesheet" href="https://docs.croct.com/assets/introduction/getting-started/style.css">
Putting everything together, your application should look like this:
1<!DOCTYPE html>2<html lang="en">3 <head>4 <meta charset="UTF-8">5 <meta name="viewport" content="width=device-width, initial-scale=1.0">6 <title>Croct | Getting Started 🚀</title>7 <link rel="shortcut icon" href="https://docs.croct.com/favicon.png"/>8 <link rel="stylesheet" href="https://docs.croct.com/assets/introduction/getting-started/style.css">9 <script src="https://cdn.croct.io/js/v1/lib/plug.js"></script>10 <script>11 // Replace with your application ID12 croct.plug({appId: 'YOUR_APPLICATION_ID'});13 </script>14 <script>15 croct.fetch('homepage-hero').then(({content}) => {16 const banner = document.getElementById('hero-banner');17 const title = banner.querySelector('h1');18 const subtitle = banner.querySelector('.subtitle');19 const cta = banner.querySelector('.cta');2021 title.textContent = content.title;22 subtitle.textContent = content.subtitle;23 cta.textContent = content.cta.label;24 cta.href = content.cta.link;25 });26 </script>27 </head>28 <body>29 <div id="hero-banner">30 <h1>Your static title</h1>31 <p class="subtitle">Your static subtitle.</p>32 <a class="cta" href="#">Your static CTA</a>33 </div>34 </body>35</html>
Opening the application in your browser, you should see something like this:
Your static subtitle.
Now, let's see how you can create a personalized experience for your users.
Personalize your application
In this final part, you will learn how to create personalized journeys. We will create a personalized experience that shows a different message based on the user's city.
Here are the steps for creating a personalized experience:
Click Experiences in the left sidebar.
Click the + New button in the center of the page.
Enter a name for your experience, such as Welcome Message.
Click Save.
After clicking Save, you will be redirected to the experience editor. Here, the first step is defining your experience's audience.
Click the + New audience button in Audiences panel.
Enter a name for your audience, such as "Users from <City name>", and an ID.
Enter the following CQL expression in the Criteria field:
Try in Playgroundlocation's city is "<City name>"Click Save.
Click Continue in the bottom right of the page to advance to the Slots step.
You can now choose which slots you want to personalize:
Click the Select slots select box, select the Homepage hero slot, and click Apply to confirm.
Click Continue in the bottom right of the page to advance to the Content step. If you see the Experiment panel, click Continue again since we are not going to cover AB testing in this guide.
The last step is defining the personalized content. In our case, we will personalize the title and subtitle for users from our target city.
In the Content step, do the following:
Click the pencil icon () on the right of the Homepage hero slot to edit its content.
Download the personalized content to save some typing again.
Click the options icon () on the top right of the Homepage hero and click the Import content button.
Select the personalized-content.json file you downloaded in the previous step.
Click Save.
The preview feature lets you see your experience before publishing it. This is especially useful for previewing an experience as an audience that your user does not belong to (i.e., someone from a different city).
You can also share the preview link with your team for feedback and review. But keep in mind that preview links are only valid for 24 hours.
Previewing an experience is straightforward:
Click the Preview button in the bottom right of the page.
Enter your application's URL in the Target URL field.
If you are developing locally, you can use the URL provided by the development server, such as http://localhost:3000.
Click Open in new tab.
Clicking Preview will open a new tab with your application. Hopefully 🤞, you will see something like this:
Now you are ready to publish your experience:
Click the chevron icon () on the right of the Preview button.
Click Publish.
After publishing your experience, you will be redirected to the Experiences dashboard where you can see several metrics about your experience.
Congrats, you have just created your first experience!
Reload your application and you should see the personalized content.
Learn more
Now that you have learned how to create a personalized experience, you can start exploring the possibilities to take your experiences to the next level.
Here are some ideas to get you started: